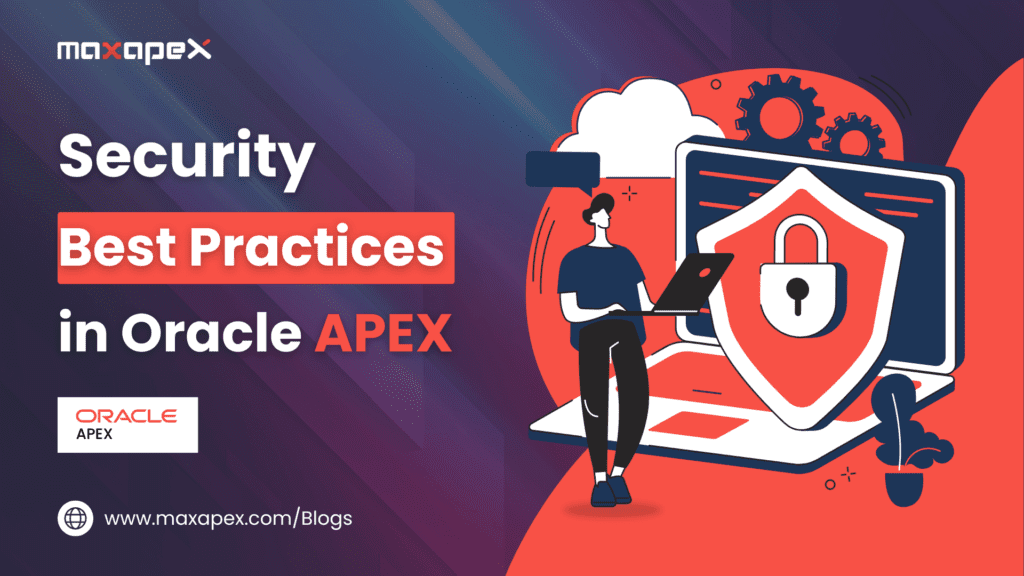
In today’s digital landscape, security is paramount. Oracle Application Express (APEX) is a powerful low-code development platform that enables developers to build scalable, secure enterprise applications with minimal effort. However, like any web application platform, it requires diligent attention to security best practices to protect sensitive data and ensure compliance with industry standards.
This guide delves into the essential security measures every Oracle APEX developer and administrator should implement to safeguard their applications.
1. Understand the Security Architecture of Oracle APEX
Practical Guidelines:
- Familiarize Yourself with APEX Architecture: Oracle APEX operates entirely within the Oracle Database. Understanding this architecture is crucial for implementing effective security measures. Study how APEX handles web requests, session management, and how it interacts with database schemas.
- Learn About Workspace Isolation: Each APEX workspace is an isolated environment. Ensure that applications are assigned to appropriate workspaces, and that user access is limited to necessary workspaces only. Regularly review workspace configurations for any anomalies.
- Understand Parsing Schemas: A parsing schema is the database schema that an APEX application uses to parse and execute SQL and PL/SQL code. Use dedicated parsing schemas for each application to limit the scope of access and reduce potential vulnerabilities.
- Review APEX Roles and Privileges: Familiarize yourself with APEX-specific roles like APEX_ADMINISTRATOR_ROLE and APEX_PUBLIC_USER. Assign these roles carefully, ensuring that users have only the privileges they need.
- Study APEX Security Features: Dive into the APEX security mechanisms, including authentication schemes, authorization schemes, and session state protection. Understanding these features will enable you to configure them effectively.
2. Implement Strong Authentication Mechanisms
Practical Guidelines:
- Choose Appropriate Authentication Schemes:
- Built-in Schemes: Use APEX’s built-in authentication schemes like Database Authentication, LDAP Directory, Social Sign-In, or Oracle Single Sign-On.
- Navigate to Shared Components > Authentication Schemes.
- Create or select an existing scheme that suits your needs.
- Custom Schemes: If built-in schemes don’t meet your requirements, develop custom authentication schemes using PL/SQL.
- Example:
- CREATE OR REPLACE FUNCTION custom_authenticate(
p_username IN VARCHAR2,
p_password IN VARCHAR2
) RETURN BOOLEAN IS
BEGIN
— Custom authentication logic here
RETURN TRUE; — or FALSE
END;
- Built-in Schemes: Use APEX’s built-in authentication schemes like Database Authentication, LDAP Directory, Social Sign-In, or Oracle Single Sign-On.
- Enforce Strong Password Policies:
- Use Oracle Database password profiles to enforce complexity, expiration, and reuse policies.
- Example:
- CREATE PROFILE secure_profile LIMIT
PASSWORD_LIFE_TIME 90
PASSWORD_REUSE_TIME 365
PASSWORD_REUSE_MAX 5
PASSWORD_VERIFY_FUNCTION verify_function;
ALTER USER apex_user PROFILE secure_profile;
- In APEX, go to Shared Components > Security Attributes and set password policies for application users.
- Use Oracle Database password profiles to enforce complexity, expiration, and reuse policies.
- Implement Multi-Factor Authentication (MFA):
- Integrate MFA through custom authentication schemes.
- Use Oracle Identity Cloud Service (IDCS) or third-party providers like Authy or Duo.
- Example:
- After primary authentication, generate and send a one-time code.
- Validate the code before granting access.
- Limit Login Attempts:
- Use APEX login throttling to prevent brute-force attacks.
- In Shared Components > Security Attributes, configure Maximum Login Failures.
- Set up account lockout policies to temporarily disable accounts after repeated failures.
- Use APEX login throttling to prevent brute-force attacks.
- Use HTTPS for Authentication Pages:
- Ensure that login pages are only accessible over HTTPS to prevent credential interception.
- Configure web server settings to enforce HTTPS connections.
MaxProtect secures your database with Comprehensive Endpoint Security, providing Robust Protection against potential threats.
3. Enforce the Principle of Least Privilege
Practical Guidelines:
- Define Specific Database Roles:
- Create roles with specific privileges required for application functionality.
- Example:
- CREATE ROLE app_user_role;
GRANT SELECT, INSERT, UPDATE ON app_schema.app_table TO app_user_role;
- Assign roles to users or schemas as needed.
- Create roles with specific privileges required for application functionality.
- Separate Schemas:
- Use one schema for application objects and another for data objects.
- This limits the exposure of data objects to application code only.
- Example:
- app_schema: Contains views, packages, and procedures.
- data_schema: Contains tables and sensitive data.
- Use one schema for application objects and another for data objects.
- Restrict Public Access:
- Revoke unnecessary privileges from the PUBLIC role.
- Example:
- REVOKE EXECUTE ON UTL_FILE FROM PUBLIC;
- Revoke unnecessary privileges from the PUBLIC role.
- Limit APEX User Privileges:
- Ensure that the database user for APEX (APEX_PUBLIC_USER) has minimal required privileges.
- Regularly review and audit user privileges.
- Use Definer’s Rights and Invoker’s Rights Appropriately:
- Set PL/SQL units to use invoker’s rights (AUTHID CURRENT_USER) when necessary.
- This allows code to execute with the privileges of the invoking user.
4. Validate and Sanitize User Inputs
Practical Guidelines:
- Implement APEX Validations:
- Use APEX validations on page items to enforce input rules.
- Navigate to the page item, click on Validation, and add server-side validations.
- Examples:
- SQL Expression Validation:
- SELECT 1 FROM dual WHERE :P1_EMAIL LIKE ‘%@%.%’
- PL/SQL Function Returning Boolean:
- RETURN LENGTH(:P1_PASSWORD) >= 8;
- Use APEX validations on page items to enforce input rules.
- Use Regular Expressions:
- Apply regular expressions to validate formats like email addresses, phone numbers, or IDs.
- Example:
- Email validation pattern:
- ^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}$
- Apply this in a validation or as a constraint.
- Example:
- Apply regular expressions to validate formats like email addresses, phone numbers, or IDs.
- Sanitize Inputs in PL/SQL:
- Use the DBMS_ASSERT package to ensure inputs are safe before using them in dynamic SQL.
- Examples:
- v_table_name := DBMS_ASSERT.SIMPLE_SQL_NAME(:P1_TABLE_NAME);
- Use the DBMS_ASSERT package to ensure inputs are safe before using them in dynamic SQL.
- Implement Database Constraints:
- Use CHECK constraints to enforce data integrity at the database level.
- Example:
- ALTER TABLE employees ADD CONSTRAINT chk_salary CHECK (salary > 0);
- Use CHECK constraints to enforce data integrity at the database level.
- Avoid Exposing Sensitive Data in URLs:
- Do not pass sensitive information via GET parameters.
- Use POST requests or session variables instead.
5. Protect Against SQL Injection
Practical Guidelines:
- Always Use Bind Variables:
- Replace concatenated SQL strings with bind variables in both SQL and PL/SQL.
- Example:
- OPEN cursor_name FOR
‘SELECT * FROM employees WHERE department_id = :dept_id’ USING :P1_DEPT_ID;
- Replace concatenated SQL strings with bind variables in both SQL and PL/SQL.
- Limit Dynamic SQL:
- Use static SQL whenever possible.
- If dynamic SQL is unavoidable, ensure that inputs are validated and sanitized.
- Use Stored Procedures and Packages:
- Encapsulate database operations in stored procedures.
- This reduces direct SQL exposure and allows for centralized input validation.
- Leverage APEX Security Features:
- Enable Session State Protection to prevent unauthorized manipulation of session items.
- Go to Shared Components > Security Attributes and enable Session State Protection.
- Enable Session State Protection to prevent unauthorized manipulation of session items.
- Educate Developers:
- Train your team on the risks of SQL injection.
- Conduct code reviews focusing on SQL statements.
MaxProtect secures your database with Comprehensive Endpoint Security, providing Robust Protection against potential threats.
6. Mitigate Cross-Site Scripting (XSS)
Practical Guidelines:
- Enable Automatic Escaping:
- In reports and forms, set columns and items to escape special characters.
- For report columns, in the Column Attributes, set Escape Special Characters to Yes.
- For page items, under Security, set Escape Special Characters to Yes.
- In reports and forms, set columns and items to escape special characters.
- Use APEX Escape Functions in PL/SQL:
- When outputting dynamic content, use functions like HTF.ESCAPE_SC or APEX_ESCAPE.HTML.
- Example:
- htp.p(APEX_ESCAPE.HTML(v_user_input));
- When outputting dynamic content, use functions like HTF.ESCAPE_SC or APEX_ESCAPE.HTML.
- Implement Content Security Policy (CSP):
- Add CSP headers to control resource loading.
- In Shared Components > Security Attributes > HTTP Response Headers, add:
- Content-Security-Policy: default-src ‘self’; script-src ‘self’;
- Add CSP headers to control resource loading.
- Avoid Inline Scripts and Styles:
- Move inline JavaScript and CSS to external files or the application’s shared components.
- This helps enforce CSP and reduces XSS risks.
- Validate Uploaded Files:
- Check file types and scan for malicious content.
- Restrict uploads to necessary file types (e.g., PDF, DOCX).
7. Use HTTPS and Secure Communication Channels
Practical Guidelines:
- Install SSL/TLS Certificates:
- Obtain certificates from trusted Certificate Authorities.
- Configure your web server (Apache, Nginx, ORDS) to use the certificates.
- For ORDS with Jetty, update the jetty-https.xml configuration.
- Enforce HTTPS Connections:
- Redirect all HTTP traffic to HTTPS.
- In Apache:
- RewriteEngine On
RewriteCond %{HTTPS} !=on
RewriteRule ^/?(.*) https://%{SERVER_NAME}/$1 [R,L]
- Redirect all HTTP traffic to HTTPS.
- Set Secure Cookie Attributes:
- In APEX, set cookies with the Secure and HttpOnly flags.
- In Shared Components > Security Attributes, configure Cookie Attributes accordingly.
- In APEX, set cookies with the Secure and HttpOnly flags.
- Disable Weak Protocols:
- Configure your server to support only TLS 1.2 or higher.
- Disable SSL 2.0, SSL 3.0, and weak cipher suites.
- Use VPNs for Internal Access:
- For administrative access or internal applications, use VPNs to secure connections.
8. Manage Sessions Securely
Practical Guidelines:
- Configure Session Timeout Values:
- In Shared Components > Security Attributes > Session Management, set:
- Maximum Session Length: Total duration a session can last.
- Maximum Session Idle Time: Time after which an inactive session expires.
- Example: Set idle time to 30 minutes and maximum length to 8 hours.
- In Shared Components > Security Attributes > Session Management, set:
- Use Secure Session Cookies:
- Ensure session cookies have the Secure and HttpOnly attributes.
- Configure in Security Attributes under Cookie Attributes.
- Implement Logout Mechanism:
- Provide a logout button or link that calls APEX_UTIL.LOGOUT.
- Example URL:
- f?p=&APP_ID.:1:&SESSION.:LOGOUT::::
- Provide a logout button or link that calls APEX_UTIL.LOGOUT.
- Prevent Session Fixation:
- Ensure that session IDs are regenerated after login.
- APEX handles this by default, but verify in custom authentication schemes.
- Enable Session State Protection:
- Protect session state by setting items to Restricted where appropriate.
- This prevents users from tampering with session variables via URL or form parameters.
9. Keep Oracle APEX and Database Updated
Practical Guidelines:
- Monitor Oracle Security Alerts:
- Subscribe to Oracle’s Critical Patch Updates (CPU) notifications.
- Regularly check the Oracle Security Alerts page.
- Schedule Regular Updates:
- Plan maintenance windows for applying patches and updates.
- Test updates in a non-production environment before rollout.
- Automate Update Processes:
- Use tools like Oracle Enterprise Manager to automate patch deployment.
- Maintain scripts for consistent update procedures.
- Update Dependencies:
- Keep web servers, middleware (ORDS), and other components up to date.
- Regularly update Java, if used, to the latest secure version.
- Document Update Procedures:
- Maintain detailed documentation of update steps and configurations.
- This ensures consistency and aids in troubleshooting.
MaxProtect secures your database with Comprehensive Endpoint Security, providing Robust Protection against potential threats.
10. Audit and Monitor Application Activity
Practical Guidelines:
- Enable Standard Auditing:
- Use the AUDIT command to track activities.
- Example:
- AUDIT SELECT ON hr.employees BY ACCESS;
- Use the AUDIT command to track activities.
- Set Up Fine-Grained Auditing (FGA):
- Define audit policies for sensitive operations.
- Example:
- BEGIN
DBMS_FGA.ADD_POLICY(
object_schema => ‘HR’,
object_name => ‘EMPLOYEES’,
policy_name => ‘EMP_SELECT_POLICY’,
audit_condition => ‘salary > 100000’,
audit_column => NULL
);
END;
- Define audit policies for sensitive operations.
- Monitor APEX-Specific Logs:
- Use views like APEX_WORKSPACE_ACTIVITY_LOG and APEX_ACTIVITY_LOG.
- Create reports or dashboards to visualize activity.
- Set Up Alerts and Notifications:
- Use Oracle Enterprise Manager or custom scripts to notify administrators of unusual activities.
- Examples:
- Excessive failed login attempts.
- Sudden spikes in data access.
- Regularly Review Logs:
- Schedule routine checks of audit logs.
- Look for patterns or anomalies that may indicate security issues.
11. Secure Configuration and Deployment
Practical Guidelines:
- Disable Unnecessary Features:
- Turn off features not used by the application, like RESTful services if not needed.
- In Workspace Administration, adjust feature settings.
- Configure Error Handling:
- Use a custom error handling function to control error messages.
- Go to Shared Components > Application Definition > Error Handling.
- Example function:
- FUNCTION error_handler (
p_error IN apex_error.t_error
) RETURN apex_error.t_error_result IS
BEGIN
— Custom error processing
RETURN apex_error.result;
END;
- Use a custom error handling function to control error messages.
- Secure File Upload Directories:
- Ensure that directories used for file uploads are not web-accessible.
- Use database BLOBs to store files when possible.
- Set Appropriate File and Directory Permissions:
- Limit access to application files and directories.
- On UNIX systems, set permissions using chmod and chown.
- Harden Web Server Configuration:
- Remove default or sample pages.
- Disable unnecessary modules.
- Implement security headers:
- X-Content-Type-Options: nosniff
- X-Frame-Options: DENY
- Referrer-Policy: no-referrer
12. Educate and Train Development Teams
Practical Guidelines:
- Conduct Regular Training Sessions:
- Schedule workshops on topics like secure coding practices, APEX security features, and recent vulnerabilities.
- Develop Security Guidelines and Checklists:
- Create documentation outlining best practices.
- Use checklists during development and code reviews to ensure compliance.
- Encourage Knowledge Sharing:
- Use internal forums or meetings to share security-related insights.
- Discuss lessons learned from past incidents.
- Promote a Security Culture:
- Encourage developers to think about security from the outset.
- Recognize and reward proactive security efforts.
- Stay Updated on Security Trends:
- Subscribe to security bulletins, blogs, and forums.
- Participate in the APEX community to share and gain knowledge.
14. Conclusion
Securing Oracle APEX applications is a continuous process that involves a combination of best practices, regular updates, and vigilant monitoring. By implementing the strategies outlined in this guide, you can significantly enhance the security posture of your applications and protect sensitive data from potential threats.
Remember, security is everyone’s responsibility. Stay informed, stay vigilant, and prioritize security in every aspect of your Oracle APEX development lifecycle.
For further reading and official Oracle guidelines, refer to the Oracle APEX Security Guide.
If you have any questions or need professional assistance with securing your Oracle APEX applications, feel free to Contact Us.